So, I have been gone for a while and I just wanted to put my story out there as to why. I am not one to blog or use social media but in today’s world that will hold you back if you do not learn to adapt.
First off I wanted to talk about the new skill I learned. Just last night I created my first sprite sheet!
It is a very simple design but it helped me get through this slump I have been in and I wanted to share it with the internet just in case it could be useful to someone out there.
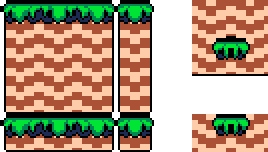

I would like to thank MortMort on YouTube for creating a great tutorial which I will link in this blog post.
Onto my second topic, my current game development situation. I, unfortunately, bit off more than I could chew. I did the exact thing that everyone from the industry said not to and I tried to create something that was way
After months of overthinking, redesigning, adding, and removing ideas from my project it became too much for me to handle and I quit working on it.
My wife has been a huge inspiration to me and without her, I would not have been able to get through that weird block type thing that was happening to me. I call it a block but I am not actually sure what was going on. What I would do is think of a mechanic to add to my game, I would work on it for a few weeks but ultimately lack the ability to execute it correctly, scrap the idea, think of a new one, and continue the cycle over and over again.
Well, the time has come for me to start this thing up again with a smaller scope which will be more in tune to the skills I currently have. I am basically Porky Pig right now in the sense of not being able to say a word because of a stutter so instead, I will say something that is close enough.
Thanks for listening to my rambling. Also, thank you for taking the time to drop by 🙂 There isn’t much here but I am changing that going forward.
See you around.